ページ
ページの作成
指定されたデータベースにページを追加します。
url = "https://api.notion.com/v1/pages"
headers = {
"accept": "application/json",
"Notion-Version": "2022-06-28",
"Authorization": f"Bearer {NOTION_TOKEN}"
}
json_data = {
# アイコンやカバーも設定可能
"icon": {
"type": "emoji",
"emoji": "?"
},
"parent": {
"type": "database_id",
"database_id": "データベースID"
},
# プロパティ
"properties": {
# タイトル
"title": {
"title": [
{
"text": {
"content": "ブログ"
}
}
]
},
},
# 本文
"children": [
# ブロック
{
"object": "block",
"heading_2": {
"rich_text": [
{
"text": {
"content": "チグサウェブ"
}
}
]
}
},
# リンク
{
"object": "block",
"paragraph": {
"rich_text": [
{
"text": {
"content": "ブログを開く",
"link": {
"url": "https://chigusa-web.com/"
}
},
"href": "https://chigusa-web.com/"
}
],
"color": "default"
}
}
]
}
response = requests.post(url, json=json_data, headers=headers)
print(response.text)
データベースにページが追加されます。
コンテンツも追加されているのが確認できます。
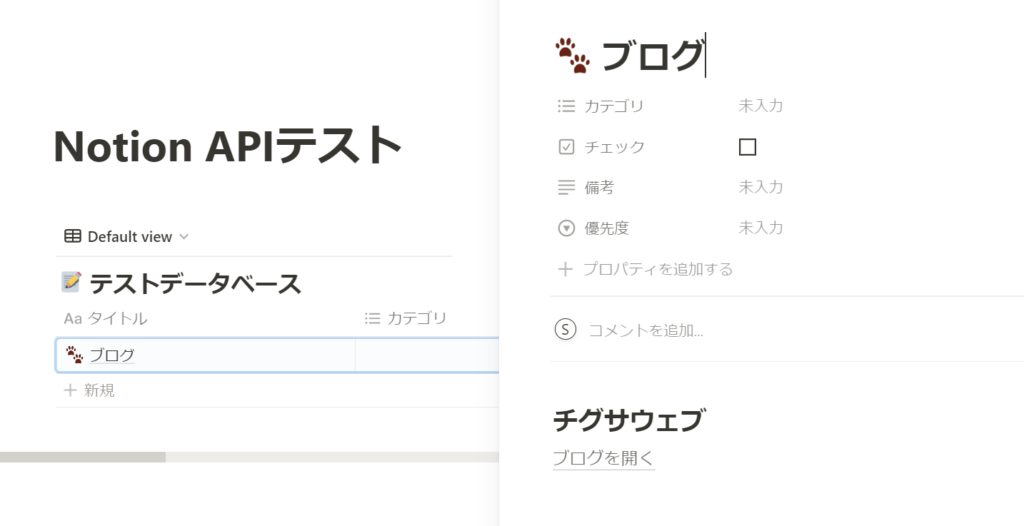
応答結果は、ページの詳細を取得できます。
{
"object": "page",
"id": "子ページID",
...
ページの情報を取得
ページIDを指定して、ページの情報を取得できます。
url = f"https://api.notion.com/v1/pages/子ページID"
headers = {
"accept": "application/json",
"Notion-Version": "2022-06-28",
"Authorization": f"Bearer {NOTION_TOKEN}"
}
response = requests.get(url, headers=headers)
print(response.text)
応答結果は、ページの詳細を取得できます。
{
"object": "page",
"id": "XXX",
"created_time": "2023-01-15T13:26:00.000Z",
"last_edited_time": "2023-01-15T13:26:00.000Z",
"created_by": {
"object": "user",
"id": "XXX"
},
"last_edited_by": {
"object": "user",
"id": "XXX"
},
"cover": null,
"icon": {
"type": "emoji",
"emoji": "?"
},
"parent": {
"type": "database_id",
"database_id": "XXX"
},
"archived": false,
"properties": {
"優先度": {
"id": "XXX",
"type": "select",
"select": null
},
"備考": {
"id": "XXX",
"type": "rich_text",
"rich_text": []
},
"チェック": {
"id": "XXX",
"type": "checkbox",
"checkbox": false
},
"カテゴリ": {
"id": "XXX",
"type": "multi_select",
"multi_select": []
},
"タイトル": {
"id": "title",
"type": "title",
"title": [
{
"type": "text",
"text": {
"content": "ブログ",
"link": null
},
"annotations": {
"bold": false,
"italic": false,
"strikethrough": false,
"underline": false,
"code": false,
"color": "default"
},
"plain_text": "ブログ",
"href": null
}
]
}
},
"url": "https://www.notion.so/XXX"
}
ブロック
ページのコンテンツを取得
ページIDを指定して、ページのコンテンツ(ブロック一覧)を取得できます。
url = f"https://api.notion.com/v1/blocks/子ページID/children"
headers = {
"accept": "application/json",
"Notion-Version": "2022-06-28",
"Authorization": f"Bearer {NOTION_TOKEN}"
}
response = requests.get(url, headers=headers)
print(response.text)
応答結果は、ページの詳細を取得できます。
{
"object": "list",
"results": [
{
"object": "block",
"id": "XXX",
"parent": {
"type": "page_id",
"page_id": "XXX"
},
"created_time": "2023-01-15T13:26:00.000Z",
"last_edited_time": "2023-01-15T13:26:00.000Z",
"created_by": {
"object": "user",
"id": "XXX"
},
"last_edited_by": {
"object": "user",
"id": "XXX"
},
"has_children": false,
"archived": false,
"type": "heading_2",
"heading_2": {
"rich_text": [
{
"type": "text",
"text": {
"content": "チグサウェブ",
"link": null
},
"annotations": {
"bold": false,
"italic": false,
"strikethrough": false,
"underline": false,
"code": false,
"color": "default"
},
"plain_text": "チグサウェブ",
"href": null
}
],
"is_toggleable": false,
"color": "default"
}
},
{
"object": "block",
"id": "XXX",
"parent": {
"type": "page_id",
"page_id": "XXX"
},
"created_time": "2023-01-15T13:26:00.000Z",
"last_edited_time": "2023-01-15T13:26:00.000Z",
"created_by": {
"object": "user",
"id": "XXX"
},
"last_edited_by": {
"object": "user",
"id": "XXX"
},
"has_children": false,
"archived": false,
"type": "paragraph",
"paragraph": {
"rich_text": [
{
"type": "text",
"text": {
"content": "ブログを開く",
"link": {
"url": "https://chigusa-web.com/"
}
},
"annotations": {
"bold": false,
"italic": false,
"strikethrough": false,
"underline": false,
"code": false,
"color": "default"
},
"plain_text": "ブログを開く",
"href": "https://chigusa-web.com/"
}
],
"color": "default"
}
}
],
"next_cursor": null,
"has_more": false,
"type": "block",
"block": {}
}
ページにブロック追加
ページにブロックを追加します。
url = f"https://api.notion.com/v1/blocks/子ページID/children"
headers = {
"accept": "application/json",
"Notion-Version": "2022-02-22",
"Authorization": f"Bearer {NOTION_TOKEN}"
}
json_data = {
"children": [
{
"heading_2": {
"rich_text": [
{
"text": {
"content": "Clibor"
}
}
]
}
},
{
"heading_3": {
"rich_text": [
{
"text": {
"content": "Windows"
}
}
]
}
},
{
"heading_3": {
"rich_text": [
{
"text": {
"content": "Mac"
}
}
]
}
},
]
}
response = requests.patch(url, json=json_data, headers=headers)
print(response.text)
ブロックが追加されました。
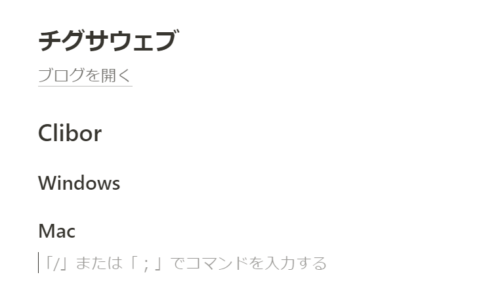
応答結果は、追加したブロックの詳細になります。
{
"object": "list",
"results": [
{
"object": "block",
"id": "子ページのブロックID",
ブロック更新
指定されたブロックを更新します。
url = f"https://api.notion.com/v1/blocks/子ページのブロックID"
headers = {
"accept": "application/json",
"Notion-Version": "2022-02-22",
"Authorization": f"Bearer {NOTION_TOKEN}"
}
json_data = {
"heading_2": {
"rich_text": [
{
"text": {
"content": "Clibor(更新)"
}
}
]
}
}
response = requests.patch(url, json=json_data, headers=headers)
print(response.text)
ブロックが更新されました。
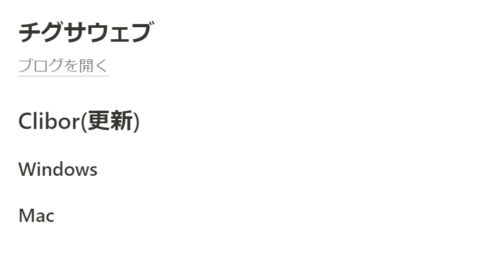
ブロック削除
指定されたブロックを削除します。
url = f"https://api.notion.com/v1/blocks/子ページのブロックID"
headers = {
"accept": "application/json",
"Notion-Version": "2022-02-22",
"Authorization": f"Bearer {NOTION_TOKEN}"
}
response = requests.delete(url, headers=headers)
print(response.text)
ブロックが削除されました。
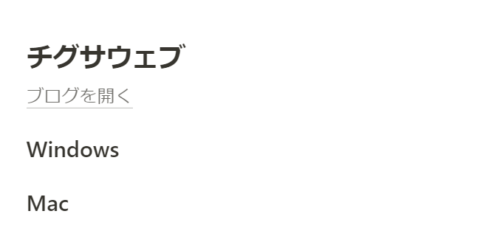
ユーザ情報
ユーザ一覧の取得
ユーザの一覧を取得することができます。
url = f"https://api.notion.com/v1/users"
headers = {
"accept": "application/json",
"Notion-Version": "2022-06-28",
"Authorization": f"Bearer {NOTION_TOKEN}"
}
response = requests.get(url, headers=headers)
print(response.text)
応答結果は、以下のようになります。
typeがbotのユーザは、APIが使用するユーザです。
{
"object": "list",
"results": [
{
"object": "user",
"id": "XXX",
"name": "XXX",
"avatar_url": null,
"type": "person",
"person": {
"email": "XXX@XXX"
}
},
{
"object": "user",
"id": "XXX",
"name": "Notion API",
"avatar_url": null,
"type": "bot",
"bot": {
"owner": {
"type": "workspace",
"workspace": true
},
"workspace_name": "APIテスト"
}
}
],
"next_cursor": null,
"has_more": false,
"type": "user",
"user": {}
}
アイディア
ざっとAPIの実装を解説しました。
データベースやページの作成、ページのブロックの作成や更新、削除などができました。
用途としては何らかのデータを、Notionのデータベースに追加し同期していく感じでしょうか。
例えば私は、Quiphaというクイズアプリを公開していますが、クイズをNotionのデータベースと連携したり、クイズの正解率などをNotionのデータベースに追加など、データの連携は考えられます。
余談ですが個人的には、ページの作成日を更新したいと思いました。
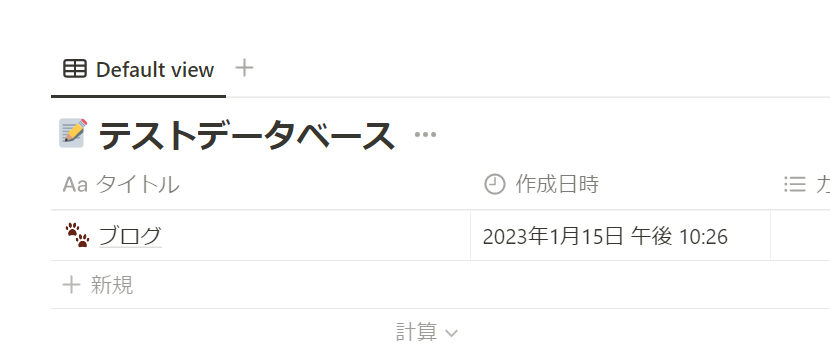
Notion上からは変更できませんが、APIならできるかも・・と思いましたが、仕様上無理でした。
無理やりAPIで更新しようとするとエラーになります。
{
"object": "error",
"status": 400,
"code": "validation_error",
"message": "Can't directly update created time"
}
また、添付ファイルの自動更新をしたいと思いました。
Evernoteは添付したファイル(エクセルなど)を、直接開いて更新した際、自動で同期を行います。
Notionは添付したファイルを一度ダウンロードし、編集後、再度アップロードし直す必要があり面倒です。
ローカルのファイルが更新されたら、Notionの指定のページの添付ファイルを更新、そういったアプリを作ろうと思いましたが、現状、NotionのAPIはファイルのアップロードに対応していませんでした。
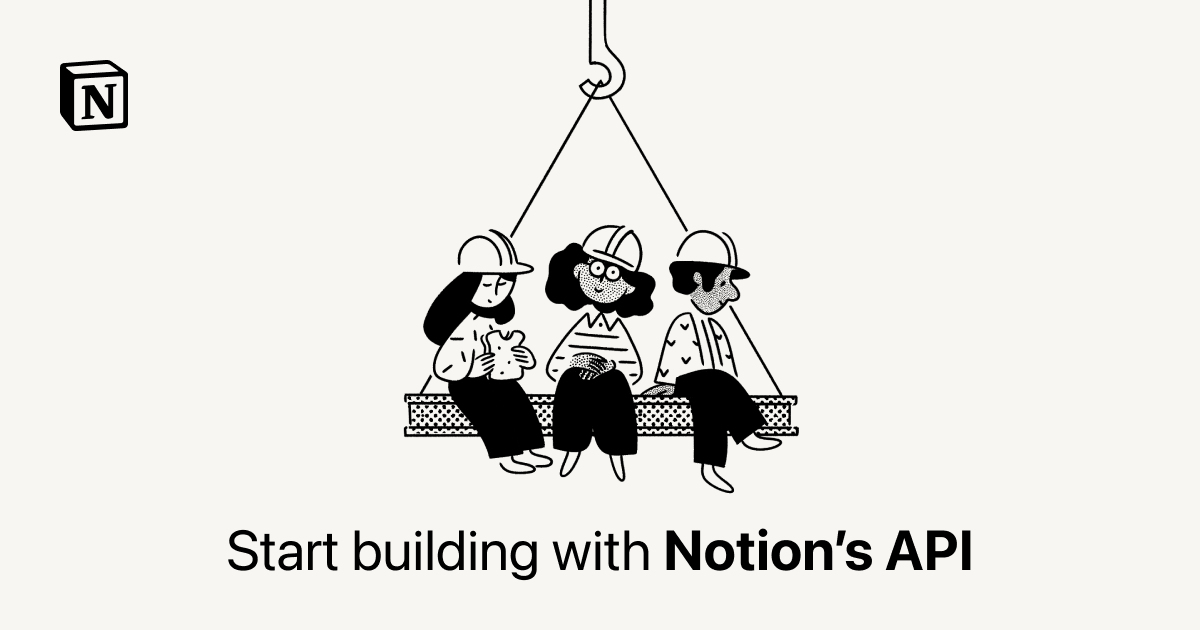
さいごに
今回は、NotionのAPIを実装して試してみました。
アイディア次第では便利な活用方法があるかもしれませんね、参考にしてみてください。
コメント